Arrow Point Towards Mouse
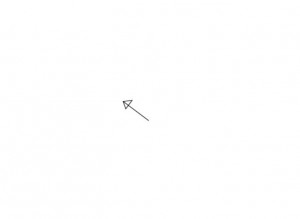
Extras : Source code
MOVE TOWARDS AN OBJECT OR POINT:
This is very similar to the above example, except in this one you need to first calculate the angle to the object or point, but of course an object has a location, so an object and a point are the same thing. You need to calculate the distance between the first object and the second object in terms of x-distance and y-distance. Now to talk about inverse trigonometry:
The 20 represents the x-distance, and the 12 represents the y-distance. That being said, the bottom-left corner represents our object, while the top-right corner represents the object we want to move our object towards. Now:
If ...
tan(θ) = (12/20)
then...
θ = tan-1(12/20)
The -1 sign means that it is the inverse form of the function. Inverse in trigonometry means that instead of using an angle in accordance with a side to get another side, you are using a side in accordance with another side to get the angle.
Just remember: for inverse tangent and for tangent, it's OPPOSITE/ADJACENT. But the thing is is that our bottom line of the triangle is facing to the right from its origin, but in order to get an accurate read of the angle we need it facing up. That being said, you flip x-distance and y-distance in the function below like such:
THE CODE:
moveAngle=Math.atan(x-distance/y-distance)*(180/Math.PI);
Now you can implement the code from the previous example and make it move in the direction "moveAngle" with whatever speed you wish.
YOU MUST UNDERSTAND:
When either side length becomes negative, if you calculate the angle with inverse tangent, the answer will be off. You can easily fix this with a few functions. You may notice when the y-distance is negative and the angle should be 120, for example, you will find it is reading as -60. Well let me tell you that whatever strange reading you get, it will always be fixable by adding either 180 or 360 to it. So, in your head try adding these values to it until you get a value between 90 and 270, because for this example, that's what we're looking for. In this case you need to add 180:
-60 + 180 = 120
You will need to add a unique value for the different conditions of where the first object is relative to the second. Look at this code:
// player is the first object
// obj is the object we want to find the angle towards
if(obj._y>player._y)
{
angle+=180;
}
if(obj._y
angle+=360;
}
if(obj._y==player._y && obj._x
angle+=360;
}
Put this code in your program and substitute the names "player" and "obj" and it will fix it. Then you will end up with a program that works, such as this demo of an arrow pointing towards the mouse:
download: arrow_point_towards_mouse.fla
http://snayflash.webs.com/trigonometry/arrow_point_towards_mouse.swf
There is an arrow on stage called "arrow". Here is the code on the stage:
onEnterFrame=function()
{
xdist=_xmouse-arrow._x;
ydist=arrow._y-_ymouse;
angle=Math.atan(xdist/ydist)*(180/Math.PI);
if(_ymouse>arrow._y)
{
angle+=180;
}
if(_ymouse
angle+=360;
}
if(_ymouse==arrow._y && _xmouse
angle+=360;
}
arrow._rotation=angle;
}
It's that simple.